Python中的多个字典操作详解
在Python中,字典是一种非常灵活且强大的数据结构,可以用来存储键值对。当我们需要处理多个字典时,Python提供了丰富的操作方法。以下是一些关于Python中多个字典操作的详细说明。
- 字典的创建与访问
在Python中,我们可以使用大括号{}
来创建字典,也可以使用dict()
函数。以下是一个简单的例子:
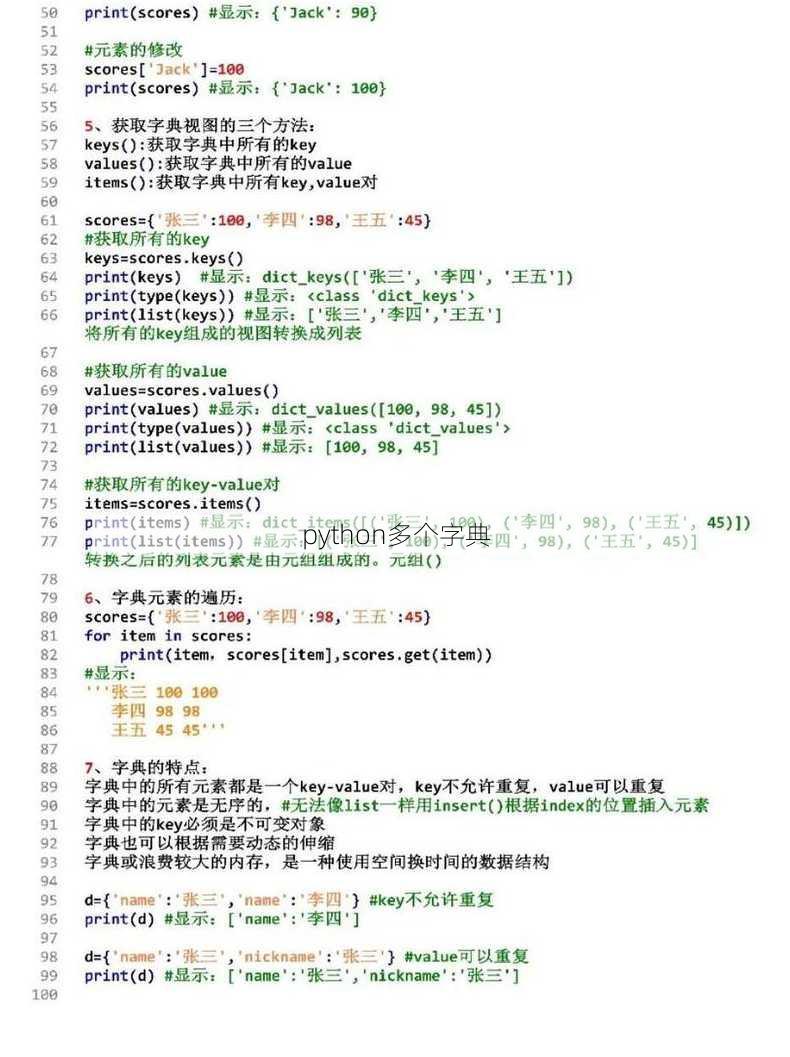
```python
dict1 {'name': 'Alice', 'age': 25}
dict2 {'name': 'Bob', 'age': 30}
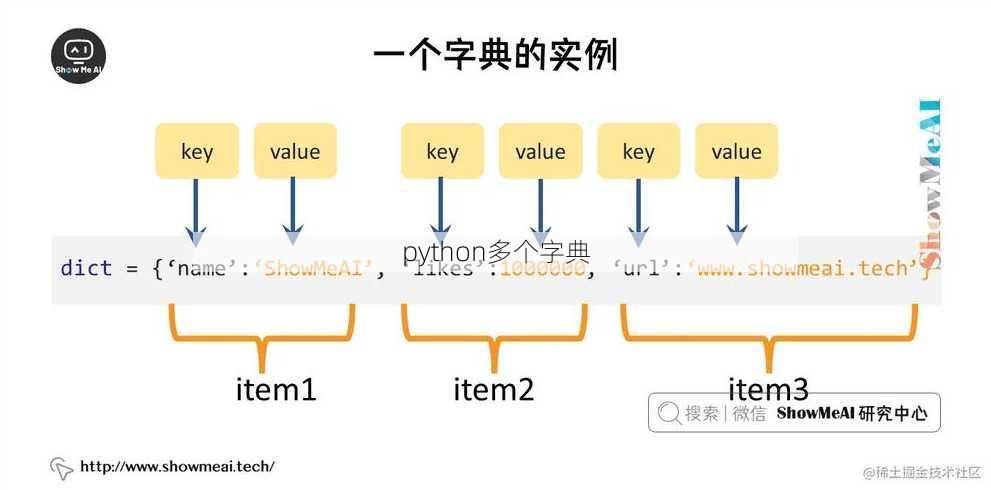
```
要访问字典中的值,我们可以使用键:
```python
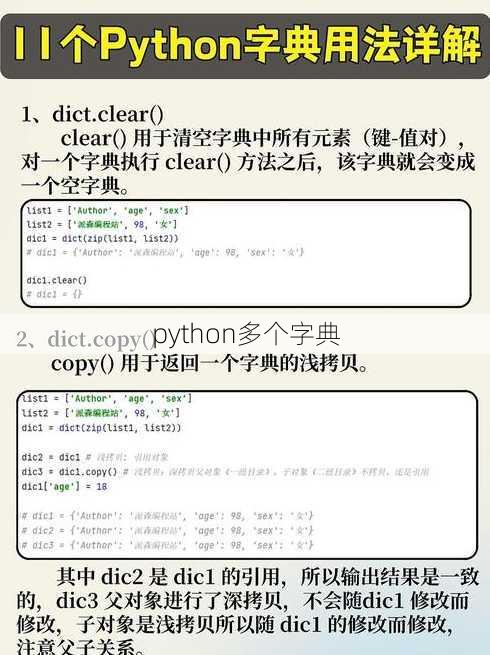
print(dict1['name']) 输出:Alice
```
- 字典的合并与更新
Python允许我们合并两个或多个字典,并更新现有的字典。以下是一些操作方法:
- 使用
update()
方法合并字典:
```python
dict1.update(dict2)
```
- 使用
|
操作符合并字典:
```python
dict1 | dict2
```
- 使用
update()
方法更新字典:
```python
dict1.update({'key': 'value'})
```
- 字典的遍历与筛选
我们可以使用循环来遍历字典,并使用条件语句来筛选出满足条件的键值对。
- 遍历字典:
```python
for key, value in dict.items():
print(key, value)
```
- 筛选字典:
```python
filtered_dict {key: value for key, value in dict.items() if value > 10}
```
真实相关问题与答案
问题1:如何合并两个字典,并保留所有键值对?
答案1: 使用update()
方法合并字典。
```python
dict1 {'name': 'Alice', 'age': 25}
dict2 {'name': 'Bob', 'age': 30}
dict1.update(dict2)
print(dict1) 输出:{'name': 'Bob', 'age': 30}
```
答案2: 使用|
操作符合并字典。
```python
dict1 {'name': 'Alice', 'age': 25}
dict2 {'name': 'Bob', 'age': 30}
dict1 | dict2
print(dict1) 输出:{'name': 'Bob', 'age': 30}
```
答案3: 使用update()
方法更新字典。
```python
dict1 {'name': 'Alice', 'age': 25}
dict2 {'name': 'Bob', 'age': 30}
dict1.update({'name': 'Bob', 'age': 30})
print(dict1) 输出:{'name': 'Bob', 'age': 30}
```
问题2:如何遍历一个字典,并打印所有键值对?
答案1: 使用for循环遍历字典。
```python
dict1 {'name': 'Alice', 'age': 25}
for key, value in dict1.items():
print(key, value)
```
答案2: 使用for循环遍历字典的键。
```python
dict1 {'name': 'Alice', 'age': 25}
for key in dict1.keys():
print(key, dict1[key])
```
答案3: 使用for循环遍历字典的值。
```python
dict1 {'name': 'Alice', 'age': 25}
for value in dict1.values():
print(value)
```
问题3:如何筛选一个字典,只保留值大于10的键值对?
答案1: 使用字典推导式筛选字典。
```python
dict1 {'name': 'Alice', 'age': 25, 'score': 8}
filtered_dict {key: value for key, value in dict1.items() if value > 10}
print(filtered_dict) 输出:{'name': 'Alice', 'age': 25}
```
答案2: 使用列表推导式筛选字典。
```python
dict1 {'name': 'Alice', 'age': 25, 'score': 8}
filtered_keys [key for key, value in dict1.items() if value > 10]
print(filtered_keys) 输出:['name', 'age']
```
答案3: 使用条件语句筛选字典。
```python
dict1 {'name': 'Alice', 'age': 25, 'score': 8}
filtered_dict {}
for key, value in dict1.items():
if value > 10:
filtered_dict[key] value
print(filtered_dict) 输出:{'name': 'Alice', 'age': 25}
```